Simple JQuery Autocomplete Using Asp Net MVC
In this tutorial I will explain how implement jQuery Autocomplete Search functionality using Asp .Net MVC and Twitter Typeahead.js library.
This is continuation to my previous post A Simple jQuery Autocomplete Search Tutorial
As I explained in my previous post Twitter Typeahead.js is An open source autocomplete JavaScript library by Twitter.It comes with rich set of features. I explained about ‘local’ and ‘prefetch’ attributes in that post.
And If you want to retrieve data from server as-you-type we have to use ‘remote’ attribute in Twitter Typeahead.js.
Open your visual studio create a simple MVC application which contains
one controller HomeController.cs and one view page Index.aspx
Add HTML Code Index.aspx view page
<asp:Content ID="indexContent" ContentPlaceHolderID="MainContent" runat="server"> <div class="CustomTemplate"> <input class="WordpressPosts typeahead" type="text" placeholder="My WordPress Posts" /> </div> </asp:Content>
And now in the same page add following jQuery Code
<link href="../../Content/examples.css" rel="stylesheet" type="text/css" /> <script type="text/javascript" src="https://twitter.github.io/typeahead.js/js/jquery-1.9.1.min.js"></script> <script type="text/javascript" src="https://twitter.github.io/typeahead.js/releases/latest/typeahead.js"></script> <script type="text/javascript" src="https://twitter.github.io/typeahead.js/js/hogan-2.0.0.js"></script> <script src="../../Content/typeahead.min.js" type="text/javascript"></script> <script type="text/javascript"> $(document).ready(function () { alert('Hello'); $('.WordpressPosts').typeahead({ name: 'Wordpress', valueKey: 'name', remote: 'Home/GetData?q=%QUERY', template: [ '<p class="repo-tag">{{tag}}</p>', '<p class="repo-name">{{name}}</p>', '<p class="repo-description">{{description}}</p>' ].join(''), engine: Hogan }); }); </script>
Everything is same as previous example except for the “remote” attribute.
remote: 'Home/GetData?q=%QUERY'
The code is straight forward we are passing queryString to a method called GetData() which return jSon data based upon input query in HomeController.
Typeahead.js ‘%QUERY’ keyword will give input search keyword which is a queryString to server.
Now we will define GetData() method in HomeController.cs file
namespace AutoComplete.Controllers { public class TagSys { public string tag { get; set; } public string name { get; set; } public string description { get; set; } public TagSys() { // // TODO: Add constructor logic here // } public List<TagSys> GetTagList() { List<TagSys> list = new List<TagSys>(); list.Add(new TagSys() { tag = "HTML5", name = "HTML5 LocalStorage API", description = "HTML5 LocalStorage API,Client Side Storage" }); list.Add(new TagSys() { tag = "HTML5", name = "HTML5 GeoLocations API", description = "HTML5 GeoLocations API,Used to Find Location" }); list.Add(new TagSys() { tag = "JavaScript", name = "JavaScript Tips And Tricks", description = "Some Useful Javascript tips and tricks" }); list.Add(new TagSys() { tag = "JavaScript", name = "JavaScript Tutorials", description = "JavaScript Tutorials" }); list.Add(new TagSys() { tag = "CSS3", name = "CSS3 Tutorials", description = "CSS3 Tutorials" }); list.Add(new TagSys() { tag = "CSS3", name = "CSS3 Animations", description = "CSS3 Animations" }); return list; } } public class HomeController : Controller { public JsonResult GetData(string q) { var list = new TagSys(); var fetchTag = list.GetTagList().Where(m => m.name.ToLower().StartsWith(q.ToLower())); return Json(fetchTag, JsonRequestBehavior.AllowGet); } } }
I defined one class called TagSys which contains tag,name,description as properties. And I added one method called GetTagList() which setups some hard coded data.
We can get the data from Database also but to simplify the code I am hard coding here. You can add your own Database Logic to retrieve data based upon queryString.
And I declared GetData() method which takes queryString as input and return jSon data based upon queryString. The Logic is straight forward no need to explain.
And the Demo will look like this.
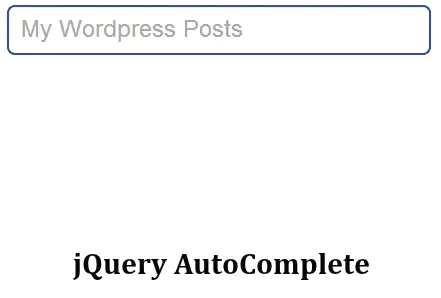
jQuery Auto Complete using Asp Net MVC
It same as previous example the difference is previous example uses ‘prefetch’ attribute which stores Json data in localStorage where as this ‘remote’ attribute return the results as-you-type from Server.
Bonus Tip: Understand C# Delegates with simple real world examples
Download the source code for jQuery autocomplete using Asp Net MVC.
I hope you have enjoyed this article.If so share this post with your friends and also join our mailing list.