HTML5 Local Storage API Tutorial With Examples
In this Tutorial I will explain all the Basics of HTML5 Local Storage API.
It’s often referred as Web Storage. Web storage is nothing but client side storage.Right now we are using cookies for the purpose of storing objects locally in client machine(in browser).
There is a big debate happening around cookie vs Local Storage I don’t want to get into that but I will start with Cookie basics, limitations of cookies and then Html5 Local Storage
Why Cookie?
Cookies are mainly used in Shopping carts to customize the user experience by storing data locally(user specific).I will explain how cookie works in high level.
- Your playing a game in online,to maintain your state of game server will store cookies in your browser.
- If you close the browser and try to access the same website then browser will send cookie along with HTTP request.
- Based on Cookie,server customize the user experience and retrieve the state of a game
- And Cookies are Domain Specific i.e., abc.com cannot access cookie of xyz.com.
Limitations of Cookies :-
- Cookies can able to store only 4k of data. For each domain browser will assign 4k of memory.
- Each time browser has to send cookie along with HTTP request,it’s inefficient especially if you are using mobile device with not a lot of bandwidth
- Difficult to handle(I mean coding) cookie key value pairs within a HTTP request.
- Each time we are sending cookie along with http request,Are we sending our personal data?
- Finally everything is handle at server side,there is no client side development.
Introduction to HTML5 Local Storage:-
In HTML5 there is no concept of Cookies everything can be done by Local Storage API.A simple JavaScript API in the browser for storing Key value pairs that are persistent and you are not limited to 4k of memory.
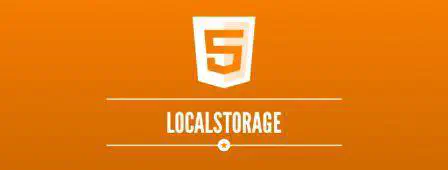
Html5 Local Storage
HTML5 Local Storage browser support:
Almost All browsers are supports HTML5 Local storage. I checked the Local storage API browser compatibility in HTML5test.com Here is the result click here.
Internet explorer 6,7 ,Opera 10 ,Firefox 3.0 are not supporting this HTML5 local storage API anyway we are not using these browsers now a days
HTML5 Local Storage limit:
All browsers today offering 5-10 MB of storage in every user’s browser.i.e., For each domain 5MB of local storage.
HTML5 local storage supports different web apps and mobile apps.Local storage is nothing but your app can store data in browser to reduce communication needed with the server.
A page can store one or more key/value pairs in the browser’s local storage.And later use key to retrieve value. These can be done by Local Storage object in local storage API.
You may thing why Local Storage why not Web Storage,Web refers to something more and we are implementing at client side,local make sense instead of Web.
Html5 Local Storage Example:-
Before going to example first we will understand basic functions of html5 local storage. With “localstorage” object we can create a key using ‘setItem‘ method.And to retrieve value use ‘getItem‘ method.
localstorage.setItem("key1","value1"); var value=localstorage.getItem("key1"); alert(value);
Most of the browsers support local storage object but safer side you can check browser compatibility as shown below
if (window["localStorage"]) { // your localStorage code here... }
And also keys are unique for example
local.storage.setItem("key1","value1"); local.storage.setItem("key1","value2"); var value = localstorage.getItem("key1"); alert(value);
Now value contain value2 not value1. And one thing Local Storage stores only string values.But in Shopping cart if we want to store item counts and prices in Local Storage you need to use parsing.
localStorage.setItem("numitems", 1); var numItems = localStorage.getItem("numitems"); var numItems = parseInt(localStorage.getItem("numitems")); numItems = numItems + 1; localStorage.setItem("numitems", numItems); localStorage.setItem("price", 9.99); var price = parseFloat(localStorage.getItem("price"));
Html5 Local Storage Array:-
Instead of using setItem and getItem methods we can use arrays as shown below
localStorage["key1"]="value1"; var value=localStorage["key1"];
And Local Storage has two interesting things :Length property and Key method.Length property holds number of items in the local store.And get can be used to retrieve value as shown below
for (var i = 0; i < localStorage.length; i++) {
var key = localStorage.key(i);
var value = localStorage[key];
alert(value);
}
or you can use foreach loop as shown below
for (var key in localStorage) {
var value = localStorage[key];alert(value); }
And you can remove keys from Local Storage using removeItem method: localStorage.removeItem(key);
Html5 Session Storage:-
If you substitute the global variable sessionStorage everywhere you’ve used localStorage then your items are stored only during the browser session. So, as soon as that session is over (in other words, the user closes the browser window), the items in storage are removed.The sessionStorage object supports exactly the same API as Local Storage.
Where we can see Local Storage keys and Values:-
And we can see the local storage key values in browsers using developer tools as shown in the pic.In developer tools select resources and Local Storage. you can select key and delete from browser itself instead of using local storage removeItem method.
To clear entire local storage you can use
localStorage.clear();
But how to use this clear method you need to create one sample HTML page.
What happens if 5MB storage exceeds:-
If 5MB storage exceeds browser should throw QUOTA_EXCEEDED_ERR exception.Most of the modern browsers will throw this exception.And remember don’t forgot to use try,catch loops.
If you are not using it may crash the browser if it exceeds 5mb storage.
Important things to remember while using Local Storage API:-
I hope you understood the basics of HTML5 Local Storage API in this Tutorial.