Matrix Effect Using HTML5 And Javascript
Now a days I am playing around with HTML5 Canvas API and Java Script. It’s an amazing option to create animations.
The Matrix movie known for its visual effects.
Then I thought is it possible to Create Matrix effects using HTML5 and JavaScript something like below.
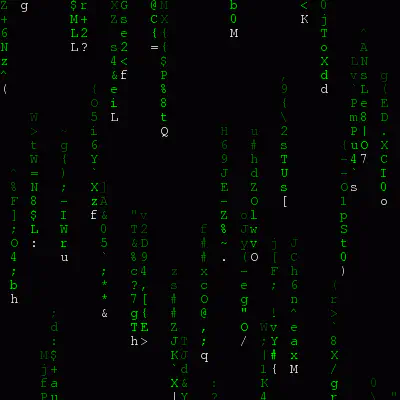
Matrix Effect
First I wrote some JavaScript code as mentioned below.
<canvas id="myCanvasMatrix" width="500" height="200" style="border:1px solid #c3c3c3;"> Please Upgrade your browser </canvas> var c1=document.getElementById("myCanvasMatrix"); var ctx1=c1.getContext("2d"); var Matrix=function(){ ctx1.fillStyle='rgba(0,0,0,.05)'; ctx1.fillRect(0,0,500,500); ctx1.fillStyle="#0f0"; ctx1.fillText('Matrix', Math.random()*(500), Math.random()*(500)); }; setInterval(Matrix,30);
I wrote one Matrix function In which I am filling canvas with “Matrix” word at random locations using Canvas fillText method(Line 10). And I am calling that function using Javascript setInterval Method (Line 12)(Interval Time 30.)
Line 7 and 8 will fill the screen with background color black and opacity of 0.05.
When each time the Matrix function is called,Canvas fillStyle will draw the background on the screen.Because of this you can see text has different colors at different places on canvas.Some text will have green color without transparent background color. This is because there will be a new layer drawn on the screen each time the Matrix is called
Line 10 will draw “Matrix” word at random locations. I am using JavaScript Math.random() to do the same. Here is the Simple Matrix Effect Demo.
But this is not what we expected,Carefully observe the above Matrix Effect Gif Image: All alphabets are drawn along Y-Axis while X-Coordinates are constant.So I changed my code little bit as shown below.This is the key point in our Algorithm to Create Matrix effect using HTML5
<canvas id="myCanvas" width="500" height="200" style="border:1px solid #c3c3c3;"> Your browser does not support the HTML5 canvas tag. </canvas> var YPositions= Array(30).join(0).split(''); var c=document.getElementById("myCanvas"); var ctx=c.getContext("2d"); var draw=function(){ ctx.fillStyle='rgba(0,0,0,.05)'; ctx.fillRect(0,0,500,500); ctx.fillStyle="#0f0"; YPositions.map(function(y,index){ x=(index*10)+10; ctx.fillText('a', x, y); if(y>100) { YPositions[index]=0; } else { YPositions[index]=y+10; } }); }; setInterval(draw,30);
Algorithm Behind Matrix Effects using HTML5 Canvas And JavaScript:
Here I declared an array (YPositions) to store Y-Coordinates.Initially array will contain all zero’s.And Inside draw() function I am using Java script Array Map function, basically It Creates a new array with the results of calling a provided function on every element in this array.
Here is the quick Example of Java Script Array Map
var numbers = [1, 4, 9]; var roots = numbers.map(Math.sqrt); /* roots is now [1, 2, 3], numbers is still [1, 4, 9] */ /* math sqrt method called on each element */
The Array index starts at ‘0’ zero so, For X -co ordinate I am adding 10,Our X coordinates are (10,20,30,40………290)(Line 12)
And Y co-ordinates will vary from (0 to 110) If Y value > 100 I am resetting array element to ‘0’ otherwise incrementing 10.(Line 14 to 21). Here I am not using new Array I am changing existing(YPositions) array itself using Java Script Array Map function.
I am drawing ‘a’ character on canvas at (x,y) locations. (Line 13).
- i.e., on first Iteration we will draw “a” at (10,10)(20,10)(30,10)……(290,10)
- Second Iteration (10,20)(20,20)(30,20)……….(290,20) and this goes until Y >100 then we will reset the value to ‘0’
Also Read : Simple Algorithm to Draw Polygon using HTML5 Canvas
I think now you understood the Logic behind this implementation if you are still in doubt refer below image.
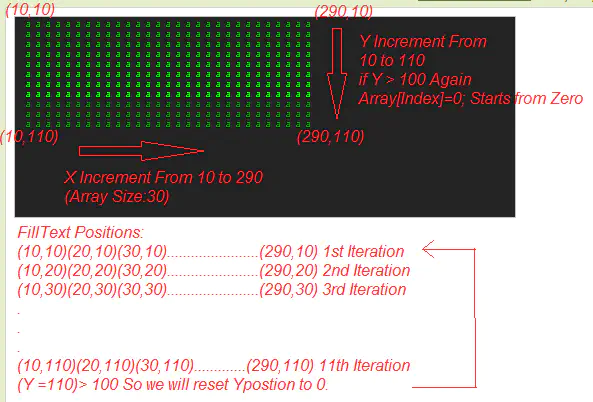
HTML5CanvasTutorial
I think now you are clear with Logic.
Now we have to replace “a” with random alphabets and Y positions to some random coordinates to create actual Matrix Effect using HTML5 and javaScript Here is the final Code.
<html><head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7.1/jquery.min.js" type="text/javascript"></script> <meta charset="utf-8"> <title>Matrix</title> </head> <body> <div align="center"> <h3>Matrix Redesigned by <i>Arunkumar Gudelli</i></h3> <canvas id="q" width="500" height="500">Sorry Browser Won't Support</canvas><br/><br/> <button id="play">Play</button> <button id="pause">pause</button> </div> <script> $(document).ready(function(){ var s=window.screen; var width = q.width=s.width; var height = q.height; var yPositions = Array(300).join(0).split(''); var ctx=q.getContext('2d'); var draw = function () { ctx.fillStyle='rgba(0,0,0,.05)'; ctx.fillRect(0,0,width,height); ctx.fillStyle='#0F0'; ctx.font = '10pt Georgia'; yPositions.map(function(y, index){ text = String.fromCharCode(1e2+Math.random()*33); x = (index * 10)+10; q.getContext('2d').fillText(text, x, y); if(y > 100 + Math.random()*1e4) { yPositions[index]=0; } else { yPositions[index] = y + 10; } }); }; RunMatrix(); function RunMatrix() { if(typeof Game_Interval != "undefined") clearInterval(Game_Interval); Game_Interval = setInterval(draw, 33); } function StopMatrix() { clearInterval(Game_Interval); } //setInterval(draw, 33); $("button#pause").click(function(){ StopMatrix();}); $("button#play").click(function(){RunMatrix();}); }) </script> </body></html>
I am using Java script String Char from Code Method(Line 27) to generate random alphabets which take ASCII Value as input and gives the corresponding character. In short, it will convert ASCII Codes to Characters.
And also I added two buttons to Play and Pause Matrix Effects.
I prefer you to read Simple Snake Game using HTML5 Canvas to understand this buttons mechanism.
Here is the Final demo of Matrix effects using HTML5 and JavaScript
Learn how to implement Google Hangout webcam Effects using HTML5 getUserMedia
I hope you’ve enjoyed this article and that it gives you more ideas on how we can implement animations using the HTML5 Canvas API.If so share this post with your friends and also join our mailing list