3 Funny Things You Can Do With C# Console
Yesterday I am discussing with one of my cousin, he is currently doing a course on C# .Net. He is experimenting lot of things in C#, I came to to 3 funny code snippets to play around C# console through him. I thought it’s a good idea to share with you people.
These code snippets may not help us in our daily life but it’s good know these things.
Fun with Console Title
Console.Title property sets the title of C# console window.
We can set animated console title like below using following code snippet.
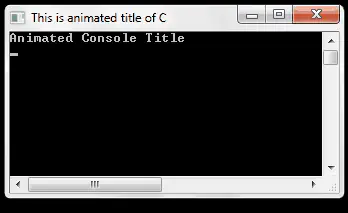
Animated Console Title
string Progressbar = "This is animated title of Console";
var title = "";
while (true)
{
for (int i = 0; i < Progressbar.Length; i++)
{
title += Progressbar[i];
Console.Title = title;
Thread.Sleep(100);
}
title = "";
}
Moving Console Window
We can use Console.SetWindowSize(numberColumns, numberRows)
property to set the console window size.
We use this property to create moving console window as shown above with following code snippet.
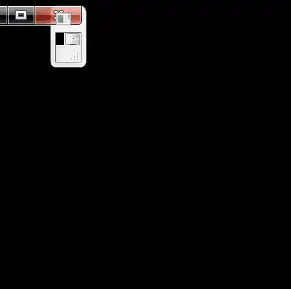
Animated Window Size Console
for (int i = 1; i < 40; i++)
{
Console.SetWindowSize(i, i);
System.Threading.Thread.Sleep(50);
}
Annoying Beep Sound
We can use Console.Beep()
method to emit beep sound from system speakers.
We can annoy the user by setting different frequencies and durations.
private static int frequency = 10000;
private static int duration = 100;
Console.WriteLine("Use keyboard arrows to adjust frequency and duration");
do
{
while (!Console.KeyAvailable)
{
Console.Beep(frequency, duration);
}
var key = Console.ReadKey(true);
switch (key.Key)
{
case ConsoleKey.UpArrow:
frequency += 100;
frequency = Math.Min(frequency, 15000);
break;
case ConsoleKey.DownArrow:
frequency -= 100;
frequency = Math.Max(frequency, 1000);
break;
case ConsoleKey.RightArrow:
duration += 100;
duration = Math.Min(duration, 1000);
break;
case ConsoleKey.LeftArrow:
duration -= 100;
duration = Math.Max(duration, 100);
break;
}
} while (true);
Bonus Tips:Understand C# Delegates and Events in C# with simple real world examples