Most Useful JavaScript Tips & Tricks For JavaScript Developers
Here I collected some JavaScript Tips & Tricks and JavaScript Best Practices which can be used in our daily projects or bookmark it for reference purpose.
This includes some useful JavaScript snippets too.
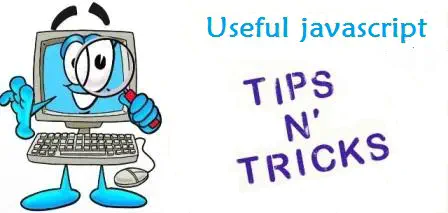
javascript tips and tricks
These JavaScript Tips And Tricks are I collected from some books and Google.
[] is better than “new Array();”:
This is from the book “JavaScript: Good Parts”.
“A common error in JavaScript programs is to use an object when an array is required or an array when an object is required. The rule is simple: when the property names are small sequential integers, you should use an array. Otherwise, use an object.”
Instead of using
var a = new Array(); a[0] = “1”; a[1] = “2”;
Use the below code snippet
var a = [‘1’,’2’];
Append an array to existing Array in JavaScript:
The following JavaScript code snippet will append array ‘a’ to ‘b’. We can use concat() method for the same but concat() method creates new array but Array Prototype push append to existing array itself
var a = [1,2,3]; var b = [4,5,6]; Array.prototype.push.apply(a, b); // a contains [1,2,3,4, 5, 6]
Check If An Object Is Array in JavaScript:
In JavaScript we can use type of to check the type of a variable but we cannot use type of for array.The below JavaScript code snippet can be used for array detection.In ECMAScript 5 we can use Array.isArray(obj) but this ECMAScript not widely adopted.
if( Object.prototype.toString.call( obj ) === '[object Array]' ) { alert( 'Array' ); }
I wrote some other code but that is not efficient as above code snippet.The concept here is the array length cannot be negative but when you are forced to do it it throws an exception.
function (obj) { try { obj.length = -1; return false; } catch (error) { return true; }
Truncate array in JavaScript:
Most of them use splice or slice method to truncate but we can use JavaScript length property to do the same. And it is more efficient than slice or splice method Here is the performance result . JavaScript slice or splice methods will return new array this might be the reason for the performance degradation
var array = ["1", "2", "3", "4", "5"]; array.length = 3; // array contains [1,2,3] var array1=array.slice(0, 3);
JavaScript array to CSV:
To convert Java Script array to CSV format comma separated values you can use below code snippet.Use JavaScript valueOf() method for comma separated values
And if you want to use pipe instead as deliminator you can use JavaScript join() method as shown below
var numbers = ['1', '2', '3', '4']; var str = numbers.valueOf(); //str: 1,2,3,4 //If you want pipe instead of comma as deliminator you can use this code var str= numbers.join("|");
If you want to convert CSV to Array use following code
var str = "1,2,3,4,5"; var numbers = str.split(","); //str[0]=1
Remove array element by Index or value in JavaScript:
If you want to remove array element by Index use the below JavaScript code snippet. We can use slice() method.
function removeElementByIndex(arr, index) { arr.splice(index, 1); } test = new Array(); test[0] = '1'; test[1] = '2'; test[2] = '3'; test[3] = '4'; test[4]='5'; //before 1,2,3,4 removeElementByIndex(test, 2); //After 1,2,4
To remove element by value use the below JavaScript code snippet
function removeElementByValue(arr, val) { for(var i=0; i<arr.length; i++) { if(arr[i] == val) { arr.splice(i, 1); break; } } }
Capture browser close Event:
To invalidate the session of an user when the user close the browser, we can use the below code snippet. In JavaScript we have events such as onbeforeunload and onunload. These events triggered whenever the page gets unload i.e., if user close the browser or move to different page.
<script language="javascript"> function PageUnloadHandler() { alert("Do something to invalidate the sessions"); } </script> <body onbeforeunload="PageUnloadHandler()"> <!-- Body content --> </body>
Redirect webpage in JavaScript:
The below JavaScript code snippet used to perform http redirect on a given URL.
window.location.href = "https://arungudelli.com";
Declare Variables Outside of the For Statement:
This is the most common mistake done by most of the developers observer the below code snippet
for(var i = 0; i < someArray.length; i++) { var container = document.getElementById('container'); container.innerHtml += 'my number: ' + i; console.log(i); }
In each iteration we must determine array length and also we should traverse through the DOM to find container instead of this assign array length and DOM elements to variables before for loop.So use the below JavaScript code snippet.
var container = document.getElementById('container'); var len = someArray.length; for(var i = 0; i < len; i++) { container.innerHtml += 'my number: ' + i; console.log(i); }
Shorten the JavaScript Code snippets:
Apart from the above code snippets and JavaScript Tips And Tricks here are the some tips to improve the code quality.
These are all “false” in boolean expressions:
null
undefined
''
or empty string- 0 the number
and below are all true:
"0"
the string[]
the empty array{}
the empty object
i.e., instead of using below code
while (x != null) {}
we can use the following JavaScript code(as long as you don’t expect x to be 0, or the empty string, or false)
while (x) {}
And to check the empty string or null we might be using this below code snippet
if (y != null && y != '') {}