3 Methods To Replace All Occurrences Of A String In JavaScript
Javascript replace() method replaces only the first occurrence of the string
To replace all occurrences of a string in Javascript, use the below methods
- Javascript string replace method with regular expression
- Javascript split and join method
- Javascript indexOf method
Take a look at the below example of Javascript Replace() method
var stringtoreplace="js"; var targetstring="vanilla js has replace method and this js method wont replace all occurrences"; targetstring.replace(stringtoreplace,"javascript"); //vanilla javascript has replace method and this js method wont replace all occurrences
It will replace only first occurrence as shown above.
On this page
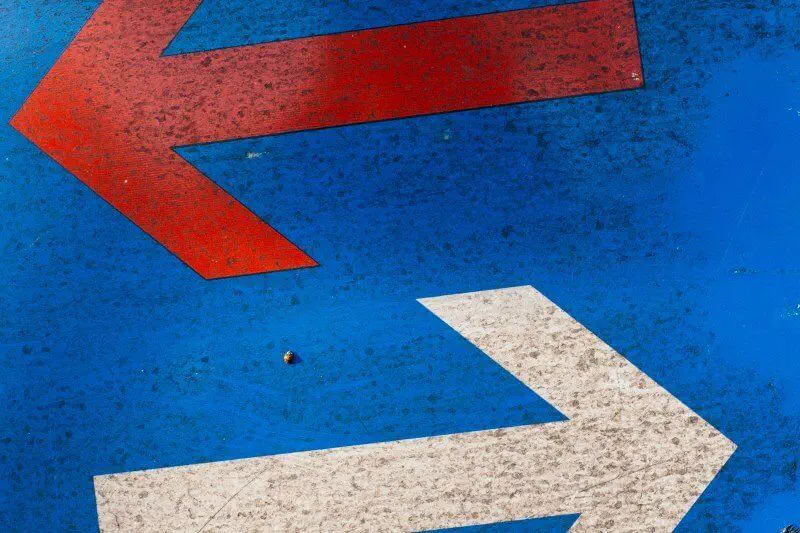
replace all occurences of a string in javascript
Replace all occurrences of a string in Javascript using replace and regex:
To replace all occurrences of a string in JavaScript, we have to use regular expressions with string replace method.
We need to pass a regular expression as a parameter with ‘g’ flag (global) instead of a normal string.
In the above example, we will pass the parameter as regular expression as shown below
targetstring.replace(/js/g,"javascript"); //vanilla javascript has replace method and this javascript method wont replace all occurrences
Or we can use the syntax of regexp as shown below to replace all occurrences
var stringReg= new RegExp(stringtoreplace, 'g'); targetstring = targetstring.replace(stringReg, 'javascript');
Depending upon our comfort we can use either of the regular expression ways.
Javascript case insensitive replace all:
The above method will not replace the case-insensitive string.
To replace all occurrences of case insensitive string in Javascript, we have to pass ‘i’ flag in addition to global flag.
Go through the below example
var target = "javascript is very popular scripting language,and It IS easy to learn"; target.replace(/is/g,''); //"javascript very popular scripting language,and It IS easy to learn"
Now we will option additional option i to the regular expression
target.replace(/is/gi,''); "javascript very popular scripting language,and It easy to learn"
Replace all occurrences of a string in Javascript using split and Join method:
Instead of this we can replace all occurrences of a string in JavaScript using split and join methods in Javascript as shown below
let some_str = 'abc def def lom abc abc def'.split('abc').join('x') console.log(some_str) //"x def def lom x x def"
At First split method returns an array of token strings, divided at the string ‘abc’ position as shown below
["", " def def lom ", " ", " def"]
And after that we will combine the string array using join method with the target string.
["", " def def lom ", " ", " def"].join('x') "x def def lom x x def"
Replace all occurrences of a string in Javascript using indexOf method:
We can write our own function to replace all string occurrences in a text using javascript indexOf method.
All we need to do is, loop through each occurrence and replace it with the given string as shown below
The below method uses indexof method to find the occurrence and replaces it using the replace method until all occurrences are completed.
function replaceAll(find, replace, str) { while( str.indexOf(find) > -1) { str = str.replace(find, replace); } return str; }
Replace all occurrences of a string with special characters:
For example, we need to replace all occurrences of a string with special characters
Take a look at the below example
var some_string = 'a?bc def def lom a?bc a?bc def';
Now we will try to replace ‘a?bc’ string with ‘x’ with the above method
some_string.replace(/a?bc/g,'x') "a?x def def lom a?x a?x def"
The string not being replaced properly why because regular expressions do not work well with special characters.
The solution for this problem is we need to escape the string which contains special character before passing it as a regular expression
Mozilla doc provided a method to escape the special characters in a string
function escapeRegExp(str) { return str.replace(/[.*+?^${}()|[\]\\]/g, "\\$&"); // $& means the whole matched string }
Now we will pass our string to the above method to escape specail characters as shown below
var stringtoreplace = escapeRegExp('a?bc') //"a\?bc"
And the we will create a regular expression using escaped string
var regextoreplace = new RegExp(stringtoreplace,'g');
Now by using the above regular expression, we will replace all occurrences of a string with special characters in the target string
some_string.replace(regextoreplace,'x'); "x def def lom x x def"
Javascript replace all commas in a string:
To replace all commas in string use the below JavaScript code
var some_string = 'abc,def,ghi'; some_string.replace(/,/g,';') "abc;def;ghi"
Javascript replace all spaces in a string:
To replace all spaces in a string use the below JavaScript code
var some_string = 'abc def ghi'; some_string.replace(/ /g,';') "abc;def;ghi"