4 Ways To Capitalize The First Letter Of A String In JavaScript
We can capitalize the first letter of a string in Javascript by using several methods as listed below
On this page
Capitalize the First Letter of a String using simple Javascript:
To capitalize the first letter of a string use the following JavaScript function. The code takes out the first letter of string & converts it into the capital letter (uppercase letter) using toUpperCase
method in JavaScript & then append it to the remaining string (uses substr()
or substring()
method in JavaScript to exclude the first letter).
Or You can use slice()
method in JavaScript to exclude the first letter.
/* * This JavaScript function takes string as input parameter * and capitalizes the first letter * @parameter : string * */ function capitalizeFirstLetter(string) { if(typeof string==undefined) return; var firstLetter = string[0] || string.charAt(0); return firstLetter ? firstLetter.toUpperCase() + string.substr(1) : ''; } function capitalizeFirstLetter(string) { if(typeof string==undefined) return; var firstLetter = string[0] || string.charAt(0); return firstLetter ? firstLetter.toUpperCase() + string.substring(1) : ''; } function capitalizeFirstLetter(string) { if(typeof string==undefined) return; var firstLetter = string[0] || string.charAt(0); return firstLetter ? firstLetter.toUpperCase() + string.slice(1) : ''; } /* Usage : * var lowercase_string = "this is test string"; * var firstLetter_capital = capitalizeFirstLetter(lowercase_string); * console.log(firstLetter_capital); * "This is test string" */
We can get the first letter of a string using string[0]
or string.charAt(0)
. string.charAt()
method is standard function & works in all browsers. The bracket version is a JavaScript and ECMAScript 5 feature. (Does not work in Internet Explorer 7).
That is why I have created one more variable called the first letter and assigning it to string[0]
or string.charAt(0)
so that it will work in all browsers.
Nowadays almost every browser supports bracket notion of accessing string (IE 8+ and Chrome, Mozilla etc)
Difference between substring(),slice() and substr()
The above code snippet uses simple JavaScript to make the first letter in a string to upper case. There are few other ways as well I will explain them one by one.
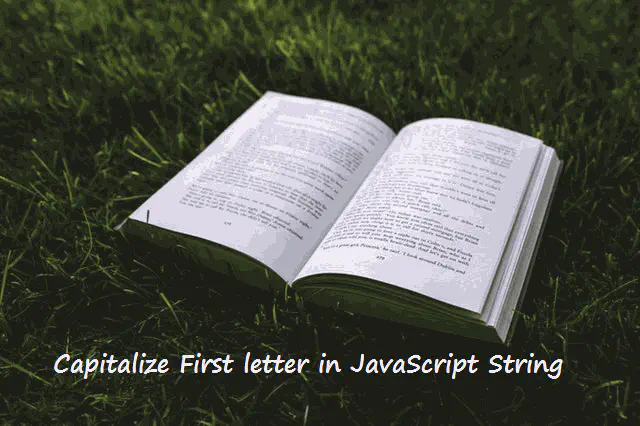
Capitalize the first letter in JavaScript String
Capitalize the First Letter of a String in JavaScript using Regular expressions:
If you are comfortable with the regular expression use the following JavaScript function to capitalize the first letter of a string.
function capitalizeFirstLetter(string) { if(typeof string==undefined) return; var firstLetter = string[0] || string.charAt(0); return firstLetter ? string.replace(/^./, firstLetter.toUpperCase()) : ''; }
But it is not required to use regular expressions for this simple operation. And the performance is very low when compared to above methods. Use Regular expressions only for complex scenarios.
Capitalize the First Letter of a String in JavaScript using ES6+(ECMAScript) features:
To capitalize the first letter of a string, use the following JavaScript ES6+ code snippet.
Here I am using Arrow functions in ES6+
const capitalizeFirstLetter = (string) => string[0] ? `${string[0].toUpperCase()}${string.substring(1)}` : ''; var lowercase_string = "this is test string"; var firstLetter_capital=capitalizeFirstLetter(lowercase_string); console.log(firstLetter_capital); //This is test string
Or We can use Destructuring assignment feature in ES6+
const capitalizeFirstLetter= ([first,...rest]) => first ? first.toUpperCase() + rest.join('') : '';
Capitalize the first letter of a string in JavaScript by Overriding String Prototype:
If you want to make the first letter of a string to uppercase frequently in your application then you can override string prototype by adding a new function
String.prototype.capitalizeFirstLetter = function() { return this.charAt(0).toUpperCase() + this.slice(1); } var lowercase_string="this is a test string"; var uppercase_string = lowercase_string.capitalizeFirstLetter(); console.log(uppercase_string); // This is a test string
Altering the String.prototype is not advisable because.
- It is very slow in terms of performance
- Maintaining the code is difficult because it is very hard to identify where the function is being added to string prototype.
- Can cause conflicts if other person uses the same name to add a new function to string prototype.
- The browser might add a new native function with the same name in future.
Best way to make the first letter of a string to uppercase in JavaScript:
If you are using the latest browser you can use ES6+ features (without Destructuring assignment) otherwise use simple JavaScript function as mentioned initially.
Regular expressions, overriding string prototype & ES6+ with Destructuring assignment are very slow in terms of performance.