How To Check If A String Is Empty/Null/Undefined In JavaScript
To check if a string is empty or null or undefined in Javascript use the following js code snippet.
var emptyString; if(!emptyString){ // String is empty }
In JavaScript, if the string is empty or null or undefined IF condition returns false.
var undefinedString; if(!undefinedString){ console.log("string is undefined"); } var emptyString=""; if(!emptyString){ console.log("string is empty"); } var nullString=null; if(!nullString){ console.log("string is null"); }
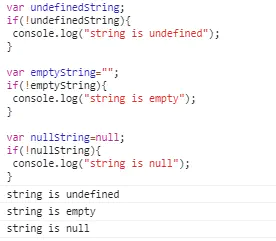
check if string is empty in JavaScript
If the string is not undefined or null and if you want to check for empty string in Javascript we can use the length property of the string prototype as shown below.
var emptyString=""; if(emptyString && emptyString.length==0){ console.log("string is empty"); }
Or you can directly check for the empty string with javascript Comparison operator “===” as shown below.
if(emptyString===""){ console.log("string is empty"); }