8 ways To Check If An Object Is Empty or not In JavaScript
Usually, we will return JSON data from server APIs (through AJAX). Sometimes the API might return an empty object i.e., “{}”.
In javascript, we can check if an object is empty or not by using
- JSON.stringify
- Object.keys (ECMA 5+)
- Object.entries (ECMA 7+)
And if you are using any third party libraries like jquery, lodash, Underscore etc you can use their existing methods for checking javascript empty object.
I will be listing down those methods in this article with examples.
On this page
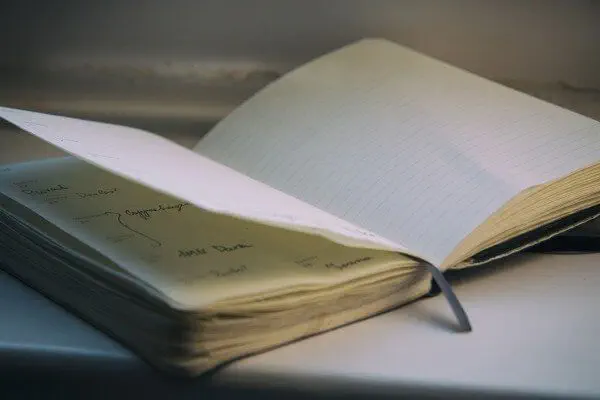
check if an object is empty javascript
Using JSON.stringify:
JSON.stringify converts an object to JSON string. We can convert the javascript empty object to JSON string and check with JSON.stringify({})
function ifObjectIsEmpty(object){ var isEmpty=true; if(JSON.stringify(object)==JSON.stringify({})){ // Object is Empty isEmpty = true; } else{ //Object is Not Empty isEmpty = false; } return isEmpty; }
Now we can pass the object to above ifObjectisEmpty method to check if an object is empty as shown below.
var emptyObject = {}; ifObjectIsEmpty(emptyObject); true // Object is Empty
Using Object.keys in es5+:
In ECMAScript 5 Object.keys() method returns an array of an object’s own property names. So to check if an object is empty or not in ES5 we can check if Object.keys().length is zero or not as shown below.
var normalObject = {a:2}; Object.keys(normalObject).length //1 var sampleObject= {}; Object.keys(sampleObject).length //0 if(Object.keys(sampleObject).length==0){ console.log('Object is empty'); }else{ console.log('Object is not empty'); }
Using Object.entries in es7+:
Object.entries() method in ECMAScript 7 returns an array of an object’s key-value pairs.
In ES7+, using Object.entries() method we can check for emptyness of an object.
var normalObject = {a:2}; Object.entries(normalObject).length //["a","2"] var sampleObject= {}; Object.entries(sampleObject).length //0 if(Object.entries(sampleObject).length==0){ console.log('Object is empty'); }else{ console.log('Object is not empty'); }
Checking if an object is empty or not in Javascript in older browsers:
The above methods might not work in older browsers like IE 7 and 8 (es5 or ECMAScript 5 and before).
So to check if an object is empty or not in all browsers we can loop through the object properties using for loop
as shown below.
function ifObjectIsEmpty(obj) { for(var prop in obj) { if(obj.hasOwnProperty(prop)) { return false; } } return JSON.stringify(obj) === JSON.stringify({}); }
Using jQuery:
In jQuery, we can use isEmptyObject() method to check if an object is empty or not as shown below
var emptyObject = {}; if(jQuery.isEmptyObject(emptyObject)){ console.log("Javscript Object is empty"); }else{ console.log("Javscript Object is not empty"); };
Using extjs:
In Extjs, we can use Ext.Object.isEmpty method to check if an object is empty or not as shown below
var emptyObject = {}; if(Ext.Object.isEmpty(emptyObject)){ console.log("Javscript Object is empty"); }else{ console.log("Javscript Object is not empty"); };
Using lodash js:
In loadash js, we can use _.isEmpty method to check if an object is empty or not as shown below
var emptyObject = {}; if(_.isEmpty(emptyObject)){ console.log("Javscript Object is empty"); }else{ console.log("Javscript Object is not empty"); };
Using Underscore js:
In Underscore js, we can use _.isEmpty method to check if an object is empty or not as shown below
var emptyObject = {}; if(_.isEmpty(emptyObject)){ console.log("Javscript Object is empty"); }else{ console.log("Javscript Object is not empty"); };