How To Create UUID/GUID In JavaScript With Examples
To create GUID or UUID in JavaScript we can use the following methods
- Javascript Math.Random()
- ES6 crypto API getRandomValues method.
GUID or UUID generated by Math.Random() may not be unique.
According to the RFC4122 version 4 complaint. A GUID or UUID should be in below format
xxxxxxxx-xxxx-Mxxx-Nxxx-xxxxxxxxxxxx Where the allowed values of M and N are 1,2,3,4 and 5
On this page
Creating GUID/UUID in Javascript using Math.Random():
To create or generate UUID or GUID in javascript use the following code with Math.Random() function
function CreateUUID() { return 'xxxxxxxx-xxxx-4xxx-yxxx-xxxxxxxxxxxx'.replace(/[xy]/g, function(c) { var r = Math.random() * 16 | 0, v = c == 'x' ? r : (r & 0x3 | 0x8); return v.toString(16); }); }
Creating GUID/UUID in Javascript using ES6 Crypto API
We can use JavaScript ES6’s crypto API to generate GUID/UUID at the client side.
crypto API comes up with a method called getRandomValues() which is used to generate a Universally Unique IDentifier as shown below
function CreateUUID() { return ([1e7]+-1e3+-4e3+-8e3+-1e11).replace(/[018]/g, c => (c ^ crypto.getRandomValues(new Uint8Array(1))[0] & 15 >> c / 4).toString(16) ) }
Generated UUID/GUID Javascript examples:
The following UUID examples are generated using the above es6 code.
CreateUUID() "95d92b5c-f19b-45d9-bbd1-759e4f2206ea" CreateUUID() "1985f93d-0cbd-4924-a821-ad425f52c49e" CreateUUID() "8b7395f7-9331-42ad-a736-3ea1aac063fa" CreateUUID() "d24da55b-c045-438f-a88e-9fa6efb08059" CreateUUID() "cc0c8f78-fcc7-43a2-ad8e-7c363da9fc24" CreateUUID() "e29fe0f5-07ea-4673-8b50-26c9323c433b" CreateUUID() "b9cba682-15a6-4444-9199-0fbbc53862a3" CreateUUID() "5d65a6a5-0449-45c3-8aa1-1aa55c0830de" CreateUUID() "141d4656-fb74-4719-bc90-ef54ba47ecbb"
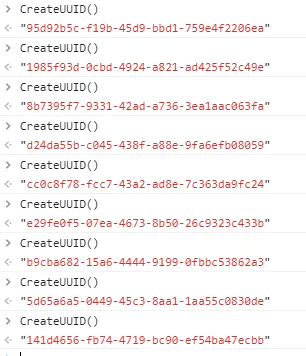
Javascript UUID GUID examples
References:
StackOverflow